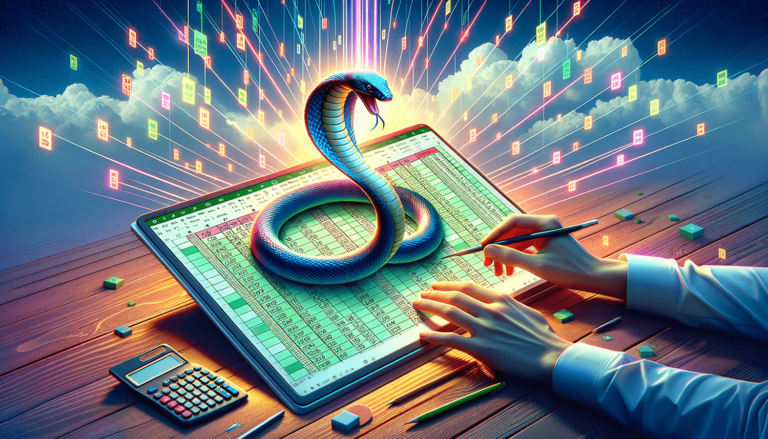
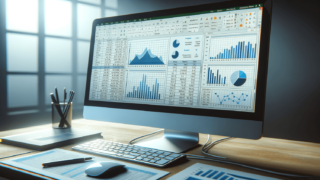
Microsoft Excel is undoubtedly one of the most widely used spreadsheet applications in the world. It is extensively used in organizations for data analysis and workflow management because of its effectiveness and efficiency. Python, on the other hand, is a popular and powerful programming language that offers great functionality and flexibility for data processing and data analysis tasks. If you work with data, you might need to use Excel files in your Python programs at some point. In this article, I will guide you on how to read Excel files in Python using efficient and effective methods that will streamline your workflow.
If you work with data, you have probably worked with an Excel file at some point. Python is a powerful programming language for data processing, machine learning, and statistical analysis. Knowing how to read Excel files in Python is essential if you want to automate data analysis tasks or integrate Python with other applications. This article provides an in-depth guide on how to read Excel files in Python, including step-by-step instructions and code snippets.
Before you start reading Excel files in Python, you should have some prior knowledge of both Python and Excel. You should also have the following installed:
You should have Python installed on your computer. You can download Python for Windows, Mac, or Linux on the official Python website.
You will need to install the openpyxl
and pandas
packages. You can easily install them using pip:
pip install openpyxl pandas
openpyxl is a Python package for working with Excel files. It allows you to read and modify Excel files using Python code. Here is how you can read an Excel file using openpyxl:
openpyxl
package:import openpyxl
workbook = openpyxl.load_workbook('example.xlsx')
sheet = workbook['Sheet1']
for row in sheet.iter_rows(): for cell in row: print(cell.value)
pandas is a popular Python library for data manipulation and analysis. It can also be used to read Excel files. Here is how you can read an Excel file using pandas:
pandas
package:import pandas as pd
df = pd.read_excel('example.xlsx')
print(df)
In conclusion, reading Excel files in Python is a useful skill for anyone who works with data. Python provides many different libraries and packages for reading Excel files, including openpyxl and pandas. By using these libraries, you can automate data analysis tasks, integrate Python with other applications, and save time in your workflow. Happy coding!
If you only want to read data from a specific sheet and cell, you can use the following code:
openpyxl
package:import openpyxl
workbook = openpyxl.load_workbook('example.xlsx')
sheet = workbook['Sheet1']
cell = sheet['A1']
print(cell.value)
This code will print the value of cell A1 in Sheet1 of example.xlsx file. You can change the sheet and cell values as per your requirements.
Reading Excel files with multiple sheets requires a slightly different approach. You can use the following code to read all the sheets in an Excel file:
openpyxl
package:import openpyxl
workbook = openpyxl.load_workbook('example.xlsx')
sheets = workbook.sheetnames
for sheet_name in sheets: sheet = workbook[sheet_name] for row in sheet.iter_rows(): for cell in row: print(cell.value)
This code will print the contents of all the sheets in example.xlsx file.
When you read an Excel file in Python, you might encounter errors such as missing files, errors in file permissions or issues with the file itself. You can use try and except blocks to handle these errors:
try: workbook = openpyxl.load_workbook('example.xlsx')except FileNotFoundError: print('File not found.')except PermissionError: print('Permission denied.')except Exception as e: print('Error:', e)
This code will handle errors for the openpyxl.load_workbook()
function and print a relevant error message for each type of error.
In conclusion, reading Excel files in Python is a helpful and necessary skill for anyone who works with data. Python provides many libraries such as openpyxl and pandas that offer a great deal of functionality for data manipulation, and you can save time in your workflow by automating data analysis tasks using Python. Remember always to install and import the required libraries, use appropriate error handling, and understand the data you are working with. With the information provided in this article, you can now read Excel files like a pro!
Here are some common questions about reading Excel files in Python:
Yes, you can read an Excel file with Python even if you don’t have Excel installed on your computer. Python libraries such as openpyxl and pandas have built-in functions that can read Excel files without the need for Excel to be installed on your computer.
openpyxl and pandas are both Python libraries for reading Excel files. openpyxl is primarily used for manipulating Excel files, while pandas is a library for data manipulation and analysis. Both libraries provide similar functionalities but have different advantages depending on your use case.
Yes, you can read an Excel file with multiple sheets using pandas. The read_excel()
function in pandas can read all sheets in an Excel file by default. You can also specify the specific sheet(s) you want to read by passing a sheet name or sheet number as an argument.
If you have empty cells or missing data in your Excel file, you can handle them in different ways depending on your use case. For example, you can use the fillna()
function in pandas to fill empty cells with specified values. You can also filter out empty cells or rows using functions such as dropna()
.
Yes, you can write data to an Excel file using Python. The openpyxl and pandas libraries provide functions for writing and saving data to an Excel file. After reading and manipulating the data, you can use the save()
function in openpyxl or the to_excel()
function in pandas to write the data to a new Excel file.
Explore the world of Microsoft PowerPoint with LearnPowerpoint.io, where we provide tailored tutorials and valuable tips to transform your presentation skills and clarify PowerPoint for enthusiasts and professionals alike.
Your ultimate guide to mastering Microsoft Word! Dive into our extensive collection of tutorials and tips designed to make Word simple and effective for users of all skill levels.
Boost your brand's online presence with Resultris Content Marketing Subscriptions. Enjoy high-quality, on-demand content marketing services to grow your business.